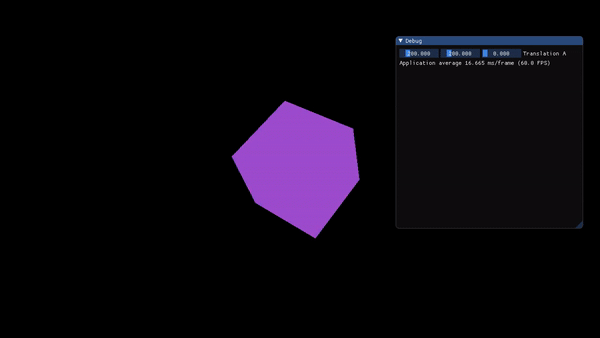
Welcome to my second DevBlog! This week as been a big week with lots of progress! Continuing from last week, I started work on rendering a voxel to the screen which required me to create a model view projection matrix that allowed me to turn the screen into 3D space by having a world space to place the object and have a view perspective(camera). The model is mapping the objects local coordinates into world space then the view from world space to camera space and lastly projection which maps from the camera to screen and combined creates the base structure of manipulating 3D space. I then enabled depth test so you would see each face correctly and they wouldn’t overlap as without enabling it a face behind may render Infront of it. I then used a set of vertices at different depths (z axis) to create the voxel you can see above! Plus, I reduced the number of vertices used to 16 by reusing vertices.
Multiple voxels
Next up on the list to do was rendering multiple voxels at once. This turned out quite easy as I just set an array of 10 vector 3 positions and using a for loop to loop through each position and drawing the voxel each time. This isn’t ideal as each draw call is very expensive as OpenGL needs to do preparations to send the data to the graphics card from the CPU to the GPU bus which is pretty slow but as for now I wanted to quickly get rendering multiple voxels on screen at once and optimise later. I was talk more in detail as to why this is not a great.
for (int i = 0; i < 10; i++)
{
glm::mat4 model = glm::translate(glm::mat4(1.0f), positions[i];
float angle = 0.0f;
model = glm::rotate(model, (float)glfwGetTime() * glm::radians(angle), glm::vec3(0.5f, 1.0f, 0.0f));
glm::mat4 mvp = proj * view * model;
shader.SetUniform4f("u_Colour", r, 0.3f, 0.8f, 1.0f);
shader.SetUniformMat4f("u_MVP", mvp);
Renderer::Draw(vertexArray, indexBuffer, shader);
}
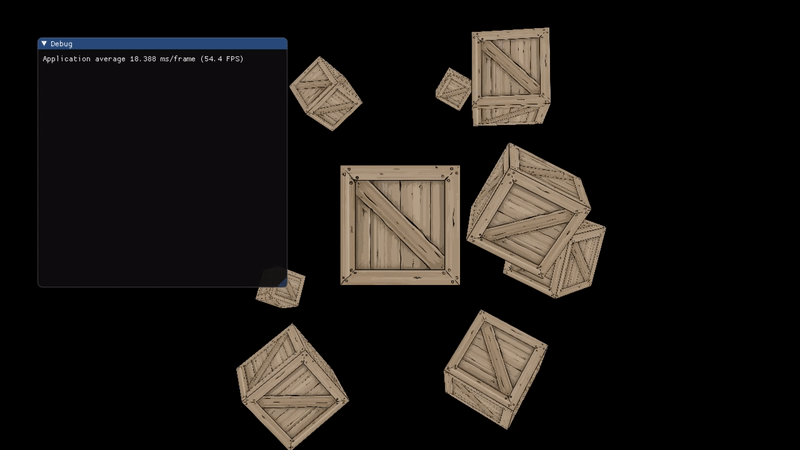
Rendering a chunk
Finally, my very first chunk rendering to the screen, why do I want to render chunks? Well instead of rendering the whole map which could be huge an impact on performance, splitting rendering into chunks and only rendering chunks of a certain distance away is much better and can be optimised a lot to get great FPS. Minecraft is a inspiration of how they render chunks and that’s what I’m aiming for. As for rendering the chunk itself it wasn’t that hard as I just done what I stated previously by rendering a block of 16x16x16 but is VERY awful as you can see in the picture, I am getting 7 fps…. With 4096 draw calls which will be the reason it is so slow but for a foundation it’s a start and I will build on it and make it a lot better.
Camera
My next goal of the week was using the view matrix to simulate a camera with free movement to move around with keyboard and mouse input as I was getting the point of needing a camera to look around in the world. I used trigonometry to get the up, forward and right direction vector to then be able to move around with WASD keys using GLFW to check if one of the keys are pressed then using delta time by getting the previous frame and current frame and subtracting then to get a constant speed of movement not dependant on the FPS. I passed the data to the view matrix and just like that it worked! I used LearnOpenGLCamera to help me get a basic camera working along with mouse input of using pitch and yaw of mouse input which gives a very nice camera movement with the mouse.
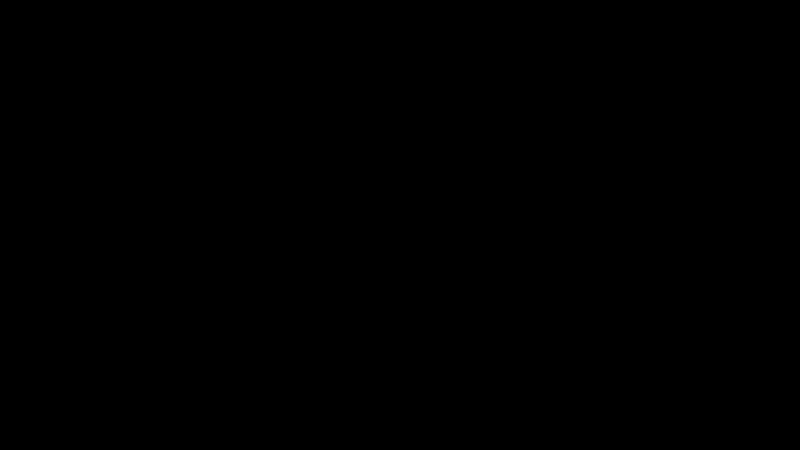
Overview
This week has been very productive as I have mostly caught up with checklist of things I want done. This week I want to focus on optimisation of rendering chunks and making the rendering chunks code stable and reusable. Now as for this week my goals are as follows:
Goals
- Use OpenGL instancing for rendering chunk
- Only render vertices that you can see
- Render a world combined of lots of chunks!
- Start researching Perlin noise and complex noise for procedural generation
Voxel Underwater Destruction!
Now with underwater destruction! pic.twitter.com/QLBIS0Y5AB
— Dennis Gustafsson (@tuxedolabs) February 29, 2020